Blog / JavaScript / Como mostrar | ocultar un campo de tipo password
Si junto a un campo de introducir contraseña, se añade un botón para mostrar su contenido, para que éste funcione sólo se debe cambiar el tipo de input.
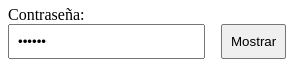
Si junto a un campo de introducir contraseña, se añade un botón para mostrar su contenido, para que éste funcione sólo se debe cambiar el tipo de input.
Para mostrar/ocultar la contraseña hay que manipular el input y el botón. Para el input cambiamos el tipo y para el botón, el texto mostrado en este caso.
<!DOCTYPE html>
<html lang="es">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
input,
button[type="button"] {
padding: 0.5rem;
}
.flex {
display: flex;
}
.form.group {
margin: 5% auto;
padding: 2rem;
}
.justify-between {
justify-content: between;
}
.mr-1 {
margin-right: 1rem;
}
</style>
</head>
<body>
<h1>Mostrar / ocultar contraseña</h1>
<div class="form-group">
<label for="password">Contraseña:</label>
<div class="flex justify-between">
<input type="password" name="password" id="password" class="mr-1" data-type="text">
<button type="button" id="toggle-password" data-text="Ocultar">Mostrar</button>
</div>
</div>
<script>
const input = document.querySelector('#password');
const toggleButton = document.querySelector('#toggle-password');
toggleButton.addEventListener('click', function (ev)
{
toggleButtonText(toggleButton);
toggleInputType(input);
});
function toggleButtonText(toggleButton, dataAttribute = 'data-text') // void
{
const textNow = toggleButton.textContent;
const textAfter = toggleButton.getAttribute(dataAttribute);
toggleButton.textContent = textAfter;
toggleButton.setAttribute(dataAttribute, textNow);
}
function toggleInputType(input, dataAttribute = 'data-type') // void
{
const typeNow = input.getAttribute('type');
const typeAfter = input.getAttribute(dataAttribute);
input.setAttribute('type', typeAfter);
input.setAttribute(dataAttribute, typeNow);
}
</script>
</body>
</html>
4-12-2024